Sometimes KR is Kind of Tricky
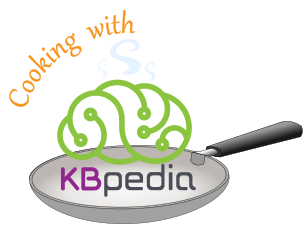
I think I pretty much lied to you about ‘roundtripping‘ and the importance of the last major section that we just completed in this Cooking with Python and KBpedia series. We had indeed accomplished the extraction-and-build cycle for classes, properties, and their annotations. These are the same roundtrip objectives we had been pursuing and doing with our Clojure procedures for years. But we were incomplete.
There is another purpose to KBpedia as a ‘scaffolding’ to external sources that is not captured in this initial ’roundtripping’ sense. If you download KBpedia, right now as version 2.50, and load it up into an editor like Protégé, you will see the KBpedia structure and its properties and annotations, but you will not see the links to the external resources that KBpedia integrates. These mappings (correspondences) are provided in separate mapping files, and have been since KBpedia was first released. This has struck me for some time as an unneeded indirection. I think the better approach is to embed these links directly into KBpedia (or whatever your master knowledge graph may be).
OK, so if we do that, does that not become part of the baseline that needs to be roundtripped? And, if to be used, what is the best practice for representing these external relationships?
Like much that has an open world approach, these kinds of questions and open-ended project requirements can stretch into the unachievable future. I don’t want to keep moving the goalposts. But I do think the role of top-level knowledge graphs is integration and coverage. The addition of key mappings to our basic expectation for our central knowledge graph seems appropriate and reasonable. Thus, for a ‘truer’ conceptualization of ’roundtripping’, I now include mappings with our basic remit.
This correction (which, of course, is an addition), and some other utilities and tools explorations, are the focus of this next major Part V in our CWPK series. Think of this next major part as wrapping our basic knowledge graph into the tools of the trade.
Representing External Resources
The standard way to handle links with an external source in most ontologies is to ‘import’ the external source. In OWL terms, this means to actually import an ontology and to incorporate the import’s full aspects. This is a bit of a more blunderbuss approach when using semantic technologies like OWL. At least in Python, as we have learned, we can actually limit our imports to specific methods or procedures.
With the standard OWL import command one brings the entire knowledge graph into the active space. Perhaps this is OK, though it does seem excessive if one only wants a small portion of the external resource, perhaps only for a specific class or predicate or three. But we have a more fundamental problem in that some of our external resources are not defined in a formal ontology subject to an import, but are just persistent URIs. Actually, with external knowledge bases like Wikipedia or Wikidata, this design is more common than one where a single ontology call can bring in all of the external structure. Moreover, even if the external source has a complete OWL-based specification, there is no guarantee that this external source embodies the same assertions and world view of our main knowledge resource, KBpedia.
If we are not bringing in a full ontology import — or may not even be able to do so because the external resources are only accessible via a URI scheme and not a logical ontology — then we will need to formulate a different linkage protocol. The one we have chosen to follow is: Give each external source its own mapping predicate, and then annotate any KBpedia resource with a mapping to show the link to the external source and its specific mapping predicate. This design decision means we can not apply a reasoner to the external sources, but that we may identify and collect them using SPARQL queries. This demarks an important realization point where we see that we can now supplement our information access and aggregation for our knowledge graph not only using logical inferencing and subsumption chains, but through directed queries that make all aspects of our information characterization, including annotations, available for selection and retrieval.
External Mapping Approach
OK, given this design, we proceed to code up our build (load) routines to bring the external mappings into KBpedia. We begin with our standard start-up instructions:
from cowpoke.__main__ import *
from cowpoke.config import *
from owlready2 import *
And then proceed to develop up our routine in the standard manner, including a header showing key configuration inputs. I explain some of the important implementation notes below the routine:
### KEY CONFIG SETTINGS (see build_deck in config.py) ###
# 'kb_src' : 'standard' # Set in master_deck
# 'loop_list' : mapping_dict.values(),
# 'base' : 'C:/1-PythonProjects/kbpedia/v300/build_ins/mappings/',
# 'ext' : '.csv',
# 'out_file' : 'C:/1-PythonProjects/kbpedia/v300/targets/ontologies/kbpedia_reference_concepts.csv',
def mapping_builder(**build_deck):
print('Beginning KBpedia mappings build . . .')
= build_deck.get('loop_list')
loop_list = build_deck.get('base')
base = build_deck.get('ext')
ext = build_deck.get('out_file')
out_file for loopval in loop_list: # Note 1
print(' . . . processing', loopval)
= (base + loopval + ext)
in_file print(in_file)
with open(in_file, 'r', encoding='utf8') as input:
= True
is_first_row = csv.DictReader(input, delimiter=',', fieldnames=['s', 'p', 'o'])
reader for row in reader: # Note 2
if is_first_row:
= False
is_first_row continue
= row['s'] # Note 3
r_s if 'kko/rc' in r_s: # Note 4
= r_s.replace('http://kbpedia.org/kko/rc/', '')
r_s = getattr(rc, r_s)
r_s else:
= r_s.replace('http://kbpedia.org/ontologies/kko#', '')
r_s = getattr(kko, r_s) # Note 5
r_s = row['p'] # Note 6
r_p = row['o']
r_o if loopval == 'dbpedia': # Note 7
= 'dbpedia_id'
kb_frag = getattr(rc, kb_frag)
kb_prop # Note 8
r_s.dbpedia_id.append(r_o) elif loopval == 'dbpedia-ontology':
= 'dbpedia_ontology_id'
kb_frag = getattr(rc, kb_frag)
kb_prop
r_s.dbpedia_ontology_id.append(r_o)elif loopval == 'geonames':
= 'geo_names_id'
kb_frag = getattr(rc, kb_frag)
kb_prop
r_s.geo_names_id.append(r_o)elif loopval == 'schema.org':
= 'schema_org_id'
kb_frag = getattr(rc, kb_frag)
kb_prop
r_s.schema_org_id.append(r_o)elif loopval == 'wikidata':
= 'wikidata_q_id'
kb_frag = getattr(rc, kb_frag)
kb_prop
r_s.wikidata_q_id.append(r_o)elif loopval == 'wikipedia':
= 'wikipedia_id'
kb_frag = getattr(rc, kb_frag)
kb_prop
r_s.wikipedia_id.append(r_o)elif loopval == 'wikipedia-categories':
= 'wikipedia_category_id'
kb_frag = getattr(rc, kb_frag)
kb_prop
r_s.wikipedia_category_id.append(r_o)else:
print(loopval, 'is not on list.')
= [r_p] # Note 9
kko.mappingAsObject[r_s, kb_prop, r_o] print(r_s)
print('External mapping uploads are complete.')
We set up our standard looping routine (1), progressing through a new ‘loop_list’ that is contained in a new dictionary (mapping_dict
) in our config.py
file. Like our other build methods, we use the csv
module and progress through the input files row-by-row (2). We pick up our column values (3) and (6) for each row, and assign them to a local variable, corresponding to the s-p-o ‘triples‘ for each row. We need to account for the fact that our KBpedia resources are under either the ‘rc’ or ‘kko’ namespaces (4), and pare them down to their fragments (since the resources are stored on file using their complete IRIs). Because these are string values we are extracting from the input files, we need to look them up and convert them to their internal attribute form (5), which are KBpedia classes in these instances.
We have a long list of switch statements (7) that detect the input file type and then pick the proper mapping predicate (there is one for each external mapping source). This long list of else-if
statements would seem to lend themselves to a helper function of some sort, but I decide just to repeat the code blocks because they are simple and straightforward. (Rather than a ‘dict’ format I want something more akin to a ‘record’ where a single key may point to many different values, but that is an expansion of the ‘dict’ approach that actually seems a little complicated in Python). All of material up to this point is really set-up and processing for the two key owlready2 algorithms that we need to use.
The first algorithm (8) we have seen before and associates the external mapped IRI to the current KBpedia class in the loop routine, and has the form of:
subject.predicate.append(object)
The only tricky part here is making sure that namespace prefixes are used as indicated and that we have converted the external mapping predicate to its internal form (kb_prop
). This code is now associating the external IRI to the subject KBpedia class. However, we are still lacking the nature of the mapping predicate.
For this purpose, we need to “annotate the annotation”, or basically reify the annotation with its specific mapping predicate. There is a format in owlready2 where we basically treat the entire tuple of the s-p-o object as the target for the added annotation, shown in the form as (9). The predicate for this purpose is the kko.mappingAsObject
. The basic format is:
kko.mappingAsObject[s, p, o] = 'specific mapping predicate'
The ‘specific mapping predicate’ is either owl:equivalentClass
, rdfs:subClassOf
, kko:superClassOf
, kko:isAbout
, or kko:isRelatedTo
. The kko:mappingAsObject
is named as it is because this specifically assigned mapping predicate applies to the external IRI when it appears in the object slot of the s-p-o triple. In other words, the triple so formed has the s-p-o construction of:
Kbpedia class - 'specific mapping predicate' - external IRI
Just as it appears in our external mapping files. It is important to keep this ordering straight, since reversing the order of the subject and object causes the inverse properties to be required (rdfs:subClassOf
is the inverse of kko:superClassOf
, kko:isAbout
is the inverse of kko:isRelatedTo
).
So, with this code block now set, we can run our routine:
**build_deck) mapping_builder(
Once processed, here is the form the mapping takes within the Protégé editor:
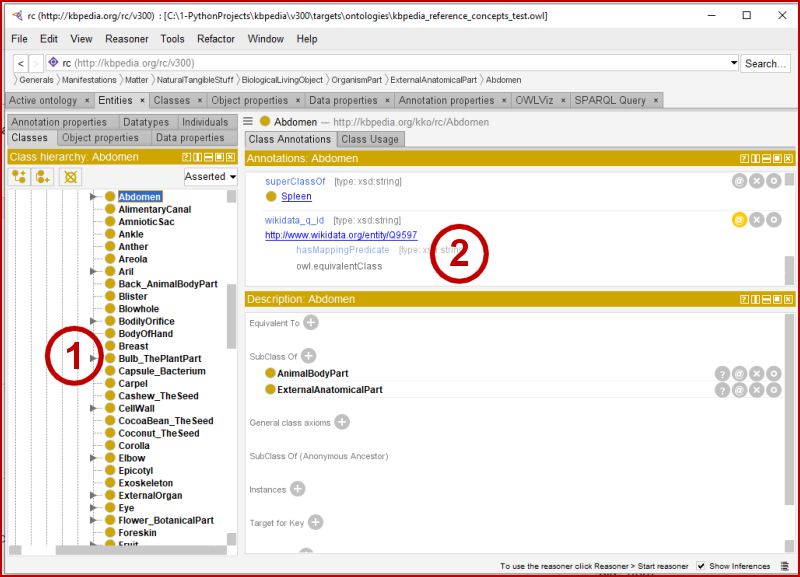
The first callout (1) indicates we successfully changed our hyphens to underscores, as discussed in the last major part. The second callout (2) shows how our mapping annotations now appear, with the annotation itself being annotated with the specific mapping predicate used.
Since we like how these mappings turned out, we decide to save the file:
'C:/1-PythonProjects/kbpedia/v300/targets/ontologies/kbpedia_reference_concepts_test.owl', format="rdfxml") kb.save(
Since these mappings add considerably to the file size, I actually save both mapped and unmapped versions for later use, depending.
Completing the Roundtrip
Now that our builds contain external mappings, it is appropriate we also create extraction routines to get these assignments back out. First, we can list all of the KBpedia RCs that have a specific external mapping (rc.dbpedia_ontology_id
in this case), which also returns a string value for the external IRI:
list(rc.dbpedia_ontology_id.get_relations())
[(rc.Person, 'http://dbpedia.org/ontology/Person'),
(rc.SoccerPlayer, 'http://dbpedia.org/ontology/SoccerPlayer'),
(rc.ConceptualWork, 'http://dbpedia.org/ontology/Work'),
(rc.Bird, 'http://dbpedia.org/ontology/Bird'),
(rc.TextualPCW, 'http://dbpedia.org/ontology/WrittenWork'),
(rc.Scientist, 'http://dbpedia.org/ontology/Scientist'),
(rc.BasketballPlayer, 'http://dbpedia.org/ontology/BasketballPlayer'),
(rc.Artist, 'http://dbpedia.org/ontology/Artist'),
(rc.GeopoliticalEntity,
'http://dbpedia.org/ontology/GeopoliticalOrganisation'),
(rc.GeopoliticalEntity, 'http://dbpedia.org/ontology/Region'),
(rc.Lighthouse, 'http://dbpedia.org/ontology/Lighthouse'),
(rc.FootballPlayer_American,
'http://dbpedia.org/ontology/GridironFootballPlayer'),
(rc.FootballPlayer_American,
'http://dbpedia.org/ontology/AmericanFootballPlayer'),
(rc.Organization, 'http://dbpedia.org/ontology/Organisation'),
(rc.Organization, 'http://dbpedia.org/ontology/EmployersOrganisation'),
(rc.Organization, 'http://dbpedia.org/ontology/OrganisationMember'),
(rc.Athlete, 'http://dbpedia.org/ontology/Athlete'),
(rc.Athlete, 'http://dbpedia.org/ontology/AthleticsPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/ArcherPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/Biathlete'),
(rc.Athlete, 'http://dbpedia.org/ontology/BobsleighAthlete'),
(rc.Athlete, 'http://dbpedia.org/ontology/Canoeist'),
(rc.Athlete, 'http://dbpedia.org/ontology/Curler'),
(rc.Athlete, 'http://dbpedia.org/ontology/DartsPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/GaelicGamesPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/HorseRider'),
(rc.Athlete,
'http://dbpedia.org/ontology/NationalCollegiateAthleticAssociationAthlete'),
(rc.Athlete, 'http://dbpedia.org/ontology/NetballPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/Rower'),
(rc.Athlete, 'http://dbpedia.org/ontology/Skater'),
(rc.Athlete, 'http://dbpedia.org/ontology/Ski-jumper'),
(rc.Athlete, 'http://dbpedia.org/ontology/SnookerChamp'),
(rc.Athlete, 'http://dbpedia.org/ontology/SnookerPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/SpeedSkater'),
(rc.Athlete, 'http://dbpedia.org/ontology/SportsTeamMember'),
(rc.Athlete, 'http://dbpedia.org/ontology/TableTennisPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/TeamMember'),
(rc.Athlete, 'http://dbpedia.org/ontology/WaterPoloPlayer'),
(rc.Athlete, 'http://dbpedia.org/ontology/WinterSportPlayer'),
(rc.Building, 'http://dbpedia.org/ontology/Building'),
(rc.Philosopher, 'http://dbpedia.org/ontology/Philosopher'),
(rc.MusicalComposition, 'http://dbpedia.org/ontology/MusicalWork'),
(rc.Horse, 'http://dbpedia.org/ontology/Horse'),
(rc.MartialArtist, 'http://dbpedia.org/ontology/MartialArtist'),
(rc.Golfer, 'http://dbpedia.org/ontology/GolfPlayer'),
(rc.Amphibian, 'http://dbpedia.org/ontology/Amphibian'),
(rc.RailroadStation, 'http://dbpedia.org/ontology/RailwayStation'),
(rc.RailroadStation, 'http://dbpedia.org/ontology/TramStation'),
(rc.Anime, 'http://dbpedia.org/ontology/Anime'),
(rc.Monument, 'http://dbpedia.org/ontology/Monument'),
(rc.Arachnid, 'http://dbpedia.org/ontology/Arachnid'),
(rc.Architect, 'http://dbpedia.org/ontology/Architect'),
(rc.Legislator, 'http://dbpedia.org/ontology/Senator'),
(rc.Article_PCW, 'http://dbpedia.org/ontology/Article'),
(rc.Cave, 'http://dbpedia.org/ontology/Cave'),
(rc.ChemicalSubstanceType, 'http://dbpedia.org/ontology/ChemicalCompound'),
(rc.ChemicalSubstanceType, 'http://dbpedia.org/ontology/ChemicalSubstance'),
(rc.TennisPlayer, 'http://dbpedia.org/ontology/TennisPlayer'),
(rc.TennisTournament, 'http://dbpedia.org/ontology/TennisTournament'),
(rc.AdministrativeDistrict,
'http://dbpedia.org/ontology/GovernmentalAdministrativeRegion'),
(rc.AdministrativeDistrict,
'http://dbpedia.org/ontology/ClericalAdministrativeRegion'),
(rc.AdministrativeDistrict, 'http://dbpedia.org/ontology/MicroRegion'),
(rc.Food, 'http://dbpedia.org/ontology/Food'),
(rc.BaseballPlayer, 'http://dbpedia.org/ontology/BaseballPlayer'),
(rc.FootballPlayer_Australian,
'http://dbpedia.org/ontology/AustralianRulesFootballPlayer'),
(rc.Municipality, 'http://dbpedia.org/ontology/Municipality'),
(rc.Municipality, 'http://dbpedia.org/ontology/FormerMunicipality'),
(rc.ElectedOfficial, 'http://dbpedia.org/ontology/OfficeHolder'),
(rc.BadmintonPlayer, 'http://dbpedia.org/ontology/BadmintonPlayer'),
(rc.PopulatedPlace, 'http://dbpedia.org/ontology/PopulatedPlace'),
(rc.RugbyPlayer, 'http://dbpedia.org/ontology/RugbyPlayer'),
(rc.School_AcademicOrganization, 'http://dbpedia.org/ontology/School'),
(rc.BodyOfWater, 'http://dbpedia.org/ontology/BodyOfWater'),
(rc.Temple, 'http://dbpedia.org/ontology/Temple'),
(rc.VolleyballPlayer, 'http://dbpedia.org/ontology/VolleyballPlayer'),
(rc.VolleyballPlayer, 'http://dbpedia.org/ontology/BeachVolleyballPlayer'),
(rc.Brewery, 'http://dbpedia.org/ontology/Brewery'),
(rc.Judge, 'http://dbpedia.org/ontology/Judge'),
(rc.Mountain, 'http://dbpedia.org/ontology/Mountain'),
(rc.Pass_Geographical, 'http://dbpedia.org/ontology/MountainPass'),
(rc.Game, 'http://dbpedia.org/ontology/Game'),
(rc.Ship, 'http://dbpedia.org/ontology/Ship'),
(rc.HotelBuilding, 'http://dbpedia.org/ontology/Hotel'),
(rc.Boxer, 'http://dbpedia.org/ontology/Boxer'),
(rc.Boxer, 'http://dbpedia.org/ontology/AmateurBoxer'),
(rc.Brain, 'http://dbpedia.org/ontology/Brain'),
(rc.Bridge, 'http://dbpedia.org/ontology/Bridge'),
(rc.Periodical, 'http://dbpedia.org/ontology/PeriodicalLiterature'),
(rc.Wrestler, 'http://dbpedia.org/ontology/Wrestler'),
(rc.VisualImage, 'http://dbpedia.org/ontology/Image'),
(rc.Professor, 'http://dbpedia.org/ontology/Professor'),
(rc.ReligiousOrganization, 'http://dbpedia.org/ontology/ClericalOrder'),
(rc.ReligiousOrganization,
'http://dbpedia.org/ontology/ReligiousOrganisation'),
(rc.Archive, 'http://dbpedia.org/ontology/Archive'),
(rc.Treaty, 'http://dbpedia.org/ontology/Treaty'),
(rc.FictionalCharacter, 'http://dbpedia.org/ontology/FictionalCharacter'),
(rc.FictionalCharacter, 'http://dbpedia.org/ontology/MythologicalFigure'),
(rc.SoftwareObject_Individual, 'http://dbpedia.org/ontology/Software'),
(rc.Parish_EcclesiasticalDistrict, 'http://dbpedia.org/ontology/Deanery'),
(rc.Actor, 'http://dbpedia.org/ontology/Actor'),
(rc.Actor, 'http://dbpedia.org/ontology/AdultActor'),
(rc.ReligiousBuilding, 'http://dbpedia.org/ontology/ReligiousBuilding'),
(rc.Concept, 'http://dbpedia.org/ontology/ScientificConcept'),
(rc.Reserve_OutdoorRegion, 'http://dbpedia.org/ontology/ProtectedArea'),
(rc.ResearchProject, 'http://dbpedia.org/ontology/ResearchProject'),
(rc.Country, 'http://dbpedia.org/ontology/Country'),
(rc.Cyclist_Athlete, 'http://dbpedia.org/ontology/Cyclist'),
(rc.Cricketer, 'http://dbpedia.org/ontology/Cricketer'),
(rc.ConstructionArtifact,
'http://dbpedia.org/ontology/ArchitecturalStructure'),
(rc.Currency, 'http://dbpedia.org/ontology/Currency'),
(rc.Graveyard, 'http://dbpedia.org/ontology/Cemetery'),
(rc.CyclingSportsEvent, 'http://dbpedia.org/ontology/CyclingCompetition'),
(rc.CyclingSportsEvent, 'http://dbpedia.org/ontology/CyclingRace'),
(rc.Racehorse, 'http://dbpedia.org/ontology/RaceHorse'),
(rc.Neighborhood, 'http://dbpedia.org/ontology/CityDistrict'),
(rc.Asteroid, 'http://dbpedia.org/ontology/Asteroid'),
(rc.City, 'http://dbpedia.org/ontology/City'),
(rc.Library_Organization, 'http://dbpedia.org/ontology/Library'),
(rc.Actor_Voice, 'http://dbpedia.org/ontology/VoiceActor'),
(rc.HumanGivenName, 'http://dbpedia.org/ontology/GivenName'),
(rc.Painter_FineArtist, 'http://dbpedia.org/ontology/Painter'),
(rc.AilmentCondition, 'http://dbpedia.org/ontology/Disease'),
(rc.Park, 'http://dbpedia.org/ontology/Park'),
(rc.EducationalOrganization,
'http://dbpedia.org/ontology/EducationalInstitution'),
(rc.EducationalOrganization, 'http://dbpedia.org/ontology/SambaSchool'),
(rc.IceHockeyPlayer, 'http://dbpedia.org/ontology/IceHockeyPlayer'),
(rc.Surfer, 'http://dbpedia.org/ontology/Surfer'),
(rc.ProteinMolecule, 'http://dbpedia.org/ontology/Protein'),
(rc.Magazine, 'http://dbpedia.org/ontology/Magazine'),
(rc.Gene_HereditaryUnit, 'http://dbpedia.org/ontology/Gene'),
(rc.Action, 'http://dbpedia.org/ontology/Activity'),
(rc.District_State_Geopolitical, 'http://dbpedia.org/ontology/District'),
(rc.Law, 'http://dbpedia.org/ontology/Law'),
(rc.FashionDesigner, 'http://dbpedia.org/ontology/FashionDesigner'),
(rc.Theater_PhysicalStructure, 'http://dbpedia.org/ontology/Theatre'),
(rc.Airport_Physical, 'http://dbpedia.org/ontology/Airport'),
(rc.PersonTypeByOccupation, 'http://dbpedia.org/ontology/CareerStation'),
(rc.PersonTypeByOccupation, 'http://dbpedia.org/ontology/Profession'),
(rc.Swimmer, 'http://dbpedia.org/ontology/Swimmer'),
(rc.Gymnast, 'http://dbpedia.org/ontology/Gymnast'),
(rc.Restaurant, 'http://dbpedia.org/ontology/Restaurant'),
(rc.Lake, 'http://dbpedia.org/ontology/Lake'),
(rc.SnowSkier, 'http://dbpedia.org/ontology/Skier'),
(rc.SnowSkier, 'http://dbpedia.org/ontology/CrossCountrySkier'),
(rc.Flag, 'http://dbpedia.org/ontology/Flag'),
(rc.Genome_GIS, 'http://dbpedia.org/ontology/GeneLocation'),
(rc.Genome_GIS, 'http://dbpedia.org/ontology/HumanGeneLocation'),
(rc.Genome_GIS, 'http://dbpedia.org/ontology/MouseGeneLocation'),
(rc.CommercialOrganization, 'http://dbpedia.org/ontology/Company'),
(rc.Photographer, 'http://dbpedia.org/ontology/Photographer'),
(rc.HistoricPeriod, 'http://dbpedia.org/ontology/HistoricalPeriod'),
(rc.HistoricPeriod, 'http://dbpedia.org/ontology/PeriodOfArtisticStyle'),
(rc.HistoricPeriod, 'http://dbpedia.org/ontology/ProtohistoricalPeriod'),
(rc.Cleric, 'http://dbpedia.org/ontology/Cleric'),
(rc.Cleric, 'http://dbpedia.org/ontology/Priest'),
(rc.Cleric, 'http://dbpedia.org/ontology/Vicar'),
(rc.MedicalFieldOfStudy, 'http://dbpedia.org/ontology/Medicine'),
(rc.PokerPlayer, 'http://dbpedia.org/ontology/PokerPlayer'),
(rc.LandTopographicalFeature, 'http://dbpedia.org/ontology/NaturalPlace'),
(rc.Glacier, 'http://dbpedia.org/ontology/Glacier'),
(rc.NonProfitOrganization,
'http://dbpedia.org/ontology/Non-ProfitOrganisation'),
(rc.NonProfitOrganization, 'http://dbpedia.org/ontology/RecordOffice'),
(rc.Mineral, 'http://dbpedia.org/ontology/Mineral'),
(rc.Diver, 'http://dbpedia.org/ontology/HighDiver'),
(rc.Fungus, 'http://dbpedia.org/ontology/Fungus'),
(rc.PersonTypeByEthnicity, 'http://dbpedia.org/ontology/EthnicGroup'),
(rc.Island, 'http://dbpedia.org/ontology/Island'),
(rc.Specification, 'http://dbpedia.org/ontology/Standard'),
(rc.FigureSkater, 'http://dbpedia.org/ontology/FigureSkater'),
(rc.Town, 'http://dbpedia.org/ontology/Town'),
(rc.FootballPlayer_Canadian,
'http://dbpedia.org/ontology/CanadianFootballPlayer'),
(rc.JournalSeries, 'http://dbpedia.org/ontology/AcademicJournal'),
(rc.JournalSeries, 'http://dbpedia.org/ontology/UndergroundJournal'),
(rc.Singer, 'http://dbpedia.org/ontology/Singer'),
(rc.Director_Movie, 'http://dbpedia.org/ontology/MovieDirector'),
(rc.ComputerGameProgram, 'http://dbpedia.org/ontology/VideoGame'),
(rc.TransportationPathSystemType,
'http://dbpedia.org/ontology/On-SiteTransportation'),
(rc.MusicalComposition_Song, 'http://dbpedia.org/ontology/Song'),
(rc.MusicalComposition_Song,
'http://dbpedia.org/ontology/EurovisionSongContestEntry'),
(rc.EukaryoticGene, 'http://dbpedia.org/ontology/HumanGene'),
(rc.EukaryoticGene, 'http://dbpedia.org/ontology/MouseGene'),
(rc.MilitaryVehicle, 'http://dbpedia.org/ontology/MilitaryVehicle'),
(rc.MusicalInstrument, 'http://dbpedia.org/ontology/Instrument'),
(rc.LacrossePlayer, 'http://dbpedia.org/ontology/LacrossePlayer'),
(rc.FashionModel, 'http://dbpedia.org/ontology/Model'),
(rc.Sculpture, 'http://dbpedia.org/ontology/Sculpture'),
(rc.MusicalComposer, 'http://dbpedia.org/ontology/MusicComposer'),
(rc.Manga, 'http://dbpedia.org/ontology/Manga'),
(rc.Astronaut, 'http://dbpedia.org/ontology/Astronaut'),
(rc.MilitaryPerson, 'http://dbpedia.org/ontology/MilitaryPerson'),
(rc.Village, 'http://dbpedia.org/ontology/Settlement'),
(rc.Village, 'http://dbpedia.org/ontology/Village'),
(rc.Village, 'http://dbpedia.org/ontology/IranSettlement'),
(rc.PublicPlace, 'http://dbpedia.org/ontology/Venue'),
(rc.Street_Generic, 'http://dbpedia.org/ontology/Street'),
(rc.PhysicalDevice, 'http://dbpedia.org/ontology/Device'),
(rc.Insect, 'http://dbpedia.org/ontology/Insect'),
(rc.SquashPlayer, 'http://dbpedia.org/ontology/SquashPlayer'),
(rc.River, 'http://dbpedia.org/ontology/River'),
(rc.Roadway, 'http://dbpedia.org/ontology/Road'),
(rc.RollerCoaster, 'http://dbpedia.org/ontology/RollerCoaster'),
(rc.TransportFacility, 'http://dbpedia.org/ontology/LaunchPad'),
(rc.TransportFacility, 'http://dbpedia.org/ontology/RouteStop'),
(rc.Volcano, 'http://dbpedia.org/ontology/Volcano'),
(rc.Dam, 'http://dbpedia.org/ontology/Dam'),
(rc.SportsTeam, 'http://dbpedia.org/ontology/SportsTeam'),
(rc.SportsTeam, 'http://dbpedia.org/ontology/CyclingTeam'),
(rc.SportsTeam, 'http://dbpedia.org/ontology/FormulaOneTeam'),
(rc.SportsTeam, 'http://dbpedia.org/ontology/HandballTeam'),
(rc.SportsTeam, 'http://dbpedia.org/ontology/NationalSoccerClub'),
(rc.SportsTeam, 'http://dbpedia.org/ontology/SpeedwayTeam'),
(rc.Election, 'http://dbpedia.org/ontology/Election'),
(rc.Monarch_HeadOfState, 'http://dbpedia.org/ontology/Monarch'),
(rc.AnimalBodyPart, 'http://dbpedia.org/ontology/AnatomicalStructure'),
(rc.BloodVessel, 'http://dbpedia.org/ontology/BloodVessel'),
(rc.PoliticalParty, 'http://dbpedia.org/ontology/PoliticalParty'),
(rc.Terminal_TransferPoint, 'http://dbpedia.org/ontology/Station'),
(rc.MilitaryAircraft, 'http://dbpedia.org/ontology/MilitaryAircraft'),
(rc.Name, 'http://dbpedia.org/ontology/Name'),
(rc.Earthquake, 'http://dbpedia.org/ontology/Earthquake'),
(rc.Automobile, 'http://dbpedia.org/ontology/Automobile'),
(rc.Animal, 'http://dbpedia.org/ontology/Animal'),
(rc.SportsEvent, 'http://dbpedia.org/ontology/SportsEvent'),
(rc.SportsEvent, 'http://dbpedia.org/ontology/FootballMatch'),
(rc.SportsEvent,
'http://dbpedia.org/ontology/InternationalFootballLeagueEvent'),
(rc.MusicSingle, 'http://dbpedia.org/ontology/Single'),
(rc.Spacecraft, 'http://dbpedia.org/ontology/Spacecraft'),
(rc.AirlineCompany, 'http://dbpedia.org/ontology/Airline'),
(rc.Bishop_Clerical, 'http://dbpedia.org/ontology/ChristianBishop'),
(rc.Engine, 'http://dbpedia.org/ontology/Engine'),
(rc.CourtCase, 'http://dbpedia.org/ontology/Case'),
(rc.CourtCase, 'http://dbpedia.org/ontology/LegalCase'),
(rc.Holiday, 'http://dbpedia.org/ontology/Holiday'),
(rc.Event, 'http://dbpedia.org/ontology/Event'),
(rc.SportsCompetition, 'http://dbpedia.org/ontology/SportCompetitionResult'),
(rc.AwardPractice, 'http://dbpedia.org/ontology/Award'),
(rc.RacingSportsEvent, 'http://dbpedia.org/ontology/Race'),
(rc.RacingSportsEvent, 'http://dbpedia.org/ontology/GrandPrix'),
(rc.Province, 'http://dbpedia.org/ontology/Province'),
(rc.Nerve, 'http://dbpedia.org/ontology/Nerve'),
(rc.Conference, 'http://dbpedia.org/ontology/AcademicConference'),
(rc.Rating, 'http://dbpedia.org/ontology/SnookerWorldRanking'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/SportsLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/AmericanFootballLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/AutoRacingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/BasketballLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/BowlingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/BoxingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/CanadianFootballLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/CricketLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/CurlingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/CyclingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/FieldHockeyLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/FormulaOneRacing'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/GolfLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/HandballLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/IceHockeyLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/InlineHockeyLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/LacrosseLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/MixedMartialArtsLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/MotorcycleRacingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/PaintballLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/PoloLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/RadioControlledRacingLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/RugbyLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/SoccerLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/SoftballLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/SpeedwayLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/TennisLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/VideogamesLeague'),
(rc.SportsLeague, 'http://dbpedia.org/ontology/VolleyballLeague'),
(rc.Competition, 'http://dbpedia.org/ontology/Competition'),
(rc.Abbey, 'http://dbpedia.org/ontology/Abbey'),
(rc.Muscle, 'http://dbpedia.org/ontology/Muscle'),
(rc.LegislativeOrganization, 'http://dbpedia.org/ontology/Legislature'),
(rc.Wine, 'http://dbpedia.org/ontology/Wine'),
(rc.SportSeason, 'http://dbpedia.org/ontology/SportsSeason'),
(rc.SportSeason, 'http://dbpedia.org/ontology/SportsTeamSeason'),
(rc.SportSeason, 'http://dbpedia.org/ontology/MotorsportSeason'),
(rc.SportSeason, 'http://dbpedia.org/ontology/NationalFootballLeagueSeason'),
(rc.SportSeason, 'http://dbpedia.org/ontology/NCAATeamSeason'),
(rc.SportSeason, 'http://dbpedia.org/ontology/SoccerClubSeason'),
(rc.SportSeason, 'http://dbpedia.org/ontology/SoccerLeagueSeason'),
(rc.HistoricalSite, 'http://dbpedia.org/ontology/HistoricPlace'),
(rc.HistoricalSite, 'http://dbpedia.org/ontology/HistoricalCountry'),
(rc.HistoricalSite, 'http://dbpedia.org/ontology/HistoricalDistrict'),
(rc.HistoricalSite, 'http://dbpedia.org/ontology/HistoricalProvince'),
(rc.HistoricalSite, 'http://dbpedia.org/ontology/HistoricalRegion'),
(rc.HistoricalSite, 'http://dbpedia.org/ontology/HistoricalSettlement'),
(rc.FieldOfStudy, 'http://dbpedia.org/ontology/AcademicSubject'),
(rc.PharmacyProductType, 'http://dbpedia.org/ontology/Drug'),
(rc.GeologicalTimePeriod, 'http://dbpedia.org/ontology/GeologicalPeriod'),
(rc.PersonWithOccupation, 'http://dbpedia.org/ontology/Deputy'),
(rc.BankOrganization, 'http://dbpedia.org/ontology/Bank'),
(rc.AutoEngine, 'http://dbpedia.org/ontology/AutomobileEngine'),
(rc.Galaxy, 'http://dbpedia.org/ontology/Galaxy'),
(rc.PowerGenerationComplex, 'http://dbpedia.org/ontology/PowerStation'),
(rc.TravelRoute, 'http://dbpedia.org/ontology/RouteOfTransportation'),
(rc.Stream, 'http://dbpedia.org/ontology/Stream'),
(rc.SportsClub, 'http://dbpedia.org/ontology/SportsClub'),
(rc.SportsClub, 'http://dbpedia.org/ontology/RugbyClub'),
(rc.SportsClub, 'http://dbpedia.org/ontology/SoccerClub'),
(rc.Sport, 'http://dbpedia.org/ontology/Sport'),
(rc.StructuredSportsEvent, 'http://dbpedia.org/ontology/PenaltyShootOut'),
(rc.SerialKiller, 'http://dbpedia.org/ontology/SerialKiller'),
(rc.GolfCourse, 'http://dbpedia.org/ontology/GolfCourse'),
(rc.Doctor_Medical, 'http://dbpedia.org/ontology/Medician'),
(rc.Canton_Swiss, 'http://dbpedia.org/ontology/Canton'),
(rc.Monarchy, 'http://dbpedia.org/ontology/Fiefdom'),
(rc.Monarchy, 'http://dbpedia.org/ontology/HistoricalAreaOfAuthority'),
(rc.Monarchy, 'http://dbpedia.org/ontology/Regency'),
(rc.Artery, 'http://dbpedia.org/ontology/Artery'),
(rc.PoliticalBeliefSystem, 'http://dbpedia.org/ontology/Ideology'),
(rc.PoliticalBeliefSystem, 'http://dbpedia.org/ontology/PoliticalConcept'),
(rc.Mammal, 'http://dbpedia.org/ontology/Mammal'),
(rc.Lawyer, 'http://dbpedia.org/ontology/Lawyer'),
(rc.Patriarch, 'http://dbpedia.org/ontology/ChristianPatriarch'),
(rc.Vodka, 'http://dbpedia.org/ontology/Vodka'),
(rc.Document, 'http://dbpedia.org/ontology/Document'),
(rc.Document, 'http://dbpedia.org/ontology/DocumentType'),
(rc.PhilosophyBeliefs, 'http://dbpedia.org/ontology/PhilosophicalConcept'),
(rc.AbstractProgrammingLanguage,
'http://dbpedia.org/ontology/ProgrammingLanguage'),
(rc.CapitalCityOfRegion, 'http://dbpedia.org/ontology/CapitalOfRegion'),
(rc.CapitalCityOfRegion, 'http://dbpedia.org/ontology/Capital'),
(rc.DomesticCat, 'http://dbpedia.org/ontology/Cat'),
(rc.Movie, 'http://dbpedia.org/ontology/Film'),
(rc.MusicalInstrumentPlayer, 'http://dbpedia.org/ontology/Instrumentalist'),
(rc.BusinessPerson, 'http://dbpedia.org/ontology/BusinessPerson'),
(rc.Enzyme, 'http://dbpedia.org/ontology/Enzyme'),
(rc.ArchitecturalTypeByStyle,
'http://dbpedia.org/ontology/ArchitecturalStructure'),
(rc.Color, 'http://dbpedia.org/ontology/Colour'),
(rc.Bacterium, 'http://dbpedia.org/ontology/Bacteria'),
(rc.Weapon, 'http://dbpedia.org/ontology/Weapon'),
(rc.Artist_Performer, 'http://dbpedia.org/ontology/BeautyQueen'),
(rc.Painting_ArtForm, 'http://dbpedia.org/ontology/Painting'),
(rc.SocialOccurrence, 'http://dbpedia.org/ontology/SocietalEvent'),
(rc.Family_Human, 'http://dbpedia.org/ontology/Family'),
(rc.FilmGenre, 'http://dbpedia.org/ontology/MovieGenre'),
(rc.Dancer_Performer, 'http://dbpedia.org/ontology/Dancer'),
(rc.Economist, 'http://dbpedia.org/ontology/Economist'),
(rc.MountainRange, 'http://dbpedia.org/ontology/MountainRange'),
(rc.Department, 'http://dbpedia.org/ontology/Department'),
(rc.Archipelago, 'http://dbpedia.org/ontology/Archipelago'),
(rc.Sea, 'http://dbpedia.org/ontology/Sea'),
(rc.WebSite, 'http://dbpedia.org/ontology/Website'),
(rc.AdultFemaleHuman, 'http://dbpedia.org/ontology/BeautyQueen'),
(rc.AthleticActivity, 'http://dbpedia.org/ontology/Athletics'),
(rc.Project, 'http://dbpedia.org/ontology/Project'),
(rc.PersonTypeByActivity, 'http://dbpedia.org/ontology/ChessPlayer'),
(rc.SociabilityBasedAction, 'http://dbpedia.org/ontology/PublicService'),
(rc.Biologist, 'http://dbpedia.org/ontology/Biologist'),
(rc.Engineer, 'http://dbpedia.org/ontology/Engineer'),
(rc.Dog, 'http://dbpedia.org/ontology/Dog'),
(rc.HumanSurname, 'http://dbpedia.org/ontology/Surname'),
(rc.Dying, 'http://dbpedia.org/ontology/Death'),
(rc.Journalist, 'http://dbpedia.org/ontology/Journalist'),
(rc.Agent_Generic, 'http://dbpedia.org/ontology/Agent'),
(rc.SocialQuantity, 'http://dbpedia.org/ontology/HumanDevelopmentIndex'),
(rc.Facility_Construct, 'http://dbpedia.org/ontology/Infrastructure'),
(rc.Crustacean, 'http://dbpedia.org/ontology/Crustacean'),
(rc.Conveying_Generic, 'http://dbpedia.org/ontology/ConveyorSystem'),
(rc.AirTransportationVehicle, 'http://dbpedia.org/ontology/Aircraft'),
(rc.TransportationDevice_Vehicle,
'http://dbpedia.org/ontology/MeanOfTransportation'),
(rc.PartOfBuilding, 'http://dbpedia.org/ontology/NoteworthyPartOfBuilding'),
(rc.PortFacility, 'http://dbpedia.org/ontology/Port'),
(rc.Driver_TransportationProfessional,
'http://dbpedia.org/ontology/MotorsportRacer'),
(rc.Driver_TransportationProfessional,
'http://dbpedia.org/ontology/RacingDriver'),
(rc.Driver_TransportationProfessional,
'http://dbpedia.org/ontology/RallyDriver'),
(rc.Fish, 'http://dbpedia.org/ontology/Fish'),
(rc.Sound, 'http://dbpedia.org/ontology/Sound'),
(rc.Album, 'http://dbpedia.org/ontology/Album'),
(rc.Drink, 'http://dbpedia.org/ontology/Beverage'),
(rc.Star, 'http://dbpedia.org/ontology/Star'),
(rc.Beer, 'http://dbpedia.org/ontology/Beer'),
(rc.Writer, 'http://dbpedia.org/ontology/Writer'),
(rc.Plant, 'http://dbpedia.org/ontology/Plant'),
(rc.God, 'http://dbpedia.org/ontology/Deity'),
(rc.ReferenceWork, 'http://dbpedia.org/ontology/Reference'),
(rc.Letter_Alphabetic, 'http://dbpedia.org/ontology/Letter'),
(rc.List_Information, 'http://dbpedia.org/ontology/List'),
(rc.MusicTypeByGenre, 'http://dbpedia.org/ontology/MusicGenre'),
(rc.RecordCompany, 'http://dbpedia.org/ontology/RecordLabel'),
(rc.Altitude, 'http://dbpedia.org/ontology/Altitude'),
(rc.Desert, 'http://dbpedia.org/ontology/Desert'),
(rc.HumanActivity, 'http://dbpedia.org/ontology/PersonFunction'),
(rc.Territory, 'http://dbpedia.org/ontology/Territory'),
(rc.Territory, 'http://dbpedia.org/ontology/OldTerritory'),
(rc.Ambassador, 'http://dbpedia.org/ontology/Ambassador'),
(rc.Moss, 'http://dbpedia.org/ontology/Moss'),
(rc.FootballTeam, 'http://dbpedia.org/ontology/AmericanFootballTeam'),
(rc.FootballTeam, 'http://dbpedia.org/ontology/AustralianFootballTeam'),
(rc.FootballTeam, 'http://dbpedia.org/ontology/CanadianFootballTeam'),
(rc.BaseballSeason, 'http://dbpedia.org/ontology/BaseballSeason'),
(rc.AmusementParkRide, 'http://dbpedia.org/ontology/AmusementParkAttraction'),
(rc.AmusementParkRide, 'http://dbpedia.org/ontology/WaterRide'),
(rc.Reptile, 'http://dbpedia.org/ontology/Reptile'),
(rc.GeopoliticalEntityBySystemOfGovernment,
'http://dbpedia.org/ontology/GovernmentType'),
(rc.VisualWork, 'http://dbpedia.org/ontology/Artwork'),
(rc.HorseRacingEvent, 'http://dbpedia.org/ontology/HorseRace'),
(rc.Forest, 'http://dbpedia.org/ontology/Forest'),
(rc.OlympicGames, 'http://dbpedia.org/ontology/Olympics'),
(rc.TownSquare, 'http://dbpedia.org/ontology/Square'),
(rc.Constellation, 'http://dbpedia.org/ontology/Constellation'),
(rc.Chancellor_HeadOfGovernment, 'http://dbpedia.org/ontology/Chancellor'),
(rc.BiologicalSpecies, 'http://dbpedia.org/ontology/Species'),
(rc.Diocese, 'http://dbpedia.org/ontology/Diocese'),
(rc.AnimalTrainer, 'http://dbpedia.org/ontology/HorseTrainer'),
(rc.TVShow, 'http://dbpedia.org/ontology/TelevisionShow'),
(rc.Club_Organization, 'http://dbpedia.org/ontology/HockeyClub'),
(rc.Valuable_PersonalPossession,
'http://dbpedia.org/ontology/CollectionOfValuables'),
(rc.Cheese, 'http://dbpedia.org/ontology/Cheese'),
(rc.Cultivar, 'http://dbpedia.org/ontology/CultivatedVariety'),
(rc.OfficialDocument, 'http://dbpedia.org/ontology/StatedResolution'),
(rc.FloralPlant, 'http://dbpedia.org/ontology/FloweringPlant'),
(rc.Conifer, 'http://dbpedia.org/ontology/Conifer'),
(rc.Archaea, 'http://dbpedia.org/ontology/Archaea'),
(rc.Archaeologist, 'http://dbpedia.org/ontology/Archeologist'),
(rc.Ocean, 'http://dbpedia.org/ontology/Ocean'),
(rc.Area, 'http://dbpedia.org/ontology/Area'),
(rc.Stadium, 'http://dbpedia.org/ontology/Arena'),
(rc.Stadium, 'http://dbpedia.org/ontology/Stadium'),
(rc.FootballSeason, 'http://dbpedia.org/ontology/FootballLeagueSeason'),
(rc.RocketPropelledTransportationDevice,
'http://dbpedia.org/ontology/Rocket'),
(rc.Aristocrat, 'http://dbpedia.org/ontology/Aristocrat'),
(rc.Aristocrat, 'http://dbpedia.org/ontology/Royalty'),
(rc.Aristocrat, 'http://dbpedia.org/ontology/BritishRoyalty'),
(rc.AristocratTypeByTitle, 'http://dbpedia.org/ontology/Noble'),
(rc.AristocraticFamilyLine, 'http://dbpedia.org/ontology/NobleFamily'),
(rc.Bone_BodyPart, 'http://dbpedia.org/ontology/Bone'),
(rc.AttackOnObject, 'http://dbpedia.org/ontology/Attack'),
(rc.MilitaryFacility, 'http://dbpedia.org/ontology/MilitaryStructure'),
(rc.Book, 'http://dbpedia.org/ontology/Book'),
(rc.Arrondissement, 'http://dbpedia.org/ontology/Arrondissement'),
(rc.MilitaryOrganization, 'http://dbpedia.org/ontology/MilitaryUnit'),
(rc.Criminal, 'http://dbpedia.org/ontology/Criminal'),
(rc.Decoration, 'http://dbpedia.org/ontology/Decoration'),
(rc.Museum_Organization, 'http://dbpedia.org/ontology/Museum'),
(rc.Language, 'http://dbpedia.org/ontology/Language'),
(rc.Community, 'http://dbpedia.org/ontology/Community'),
(rc.ArtistTypeByArtForm, 'http://dbpedia.org/ontology/ArtisticGenre'),
(rc.Band_MusicGroup, 'http://dbpedia.org/ontology/Band'),
(rc.Murderer, 'http://dbpedia.org/ontology/Murderer'),
(rc.Soccer, 'http://dbpedia.org/ontology/Soccer'),
(rc.CelestialObject, 'http://dbpedia.org/ontology/Globularswarm'),
(rc.CelestialObject, 'http://dbpedia.org/ontology/Openswarm'),
(rc.CelestialObject, 'http://dbpedia.org/ontology/Swarm'),
(rc.SpaceMission, 'http://dbpedia.org/ontology/SpaceMission'),
(rc.ClothingTypeByStyle, 'http://dbpedia.org/ontology/LineOfFashion'),
(rc.SportsPlayingArea, 'http://dbpedia.org/ontology/SportFacility'),
(rc.Atoll, 'http://dbpedia.org/ontology/Atoll'),
(rc.SalesActivity, 'http://dbpedia.org/ontology/Sales'),
(rc.ReligiousBeliefs, 'http://dbpedia.org/ontology/TheologicalConcept'),
(rc.BasketballTeam, 'http://dbpedia.org/ontology/BasketballTeam'),
(rc.AustralianFootballLeague,
'http://dbpedia.org/ontology/AustralianFootballLeague'),
(rc.CarRacing, 'http://dbpedia.org/ontology/DTMRacer'),
(rc.State_Geopolitical, 'http://dbpedia.org/ontology/State'),
(rc.Motorcycle, 'http://dbpedia.org/ontology/Motorcycle'),
(rc.TrainEngine, 'http://dbpedia.org/ontology/Locomotive'),
(rc.Gate, 'http://dbpedia.org/ontology/Gate'),
(rc.BoardGame, 'http://dbpedia.org/ontology/BoardGame'),
(rc.Tournament, 'http://dbpedia.org/ontology/Tournament'),
(rc.Bay, 'http://dbpedia.org/ontology/Bay'),
(rc.Poem, 'http://dbpedia.org/ontology/Poem'),
(rc.Baronet, 'http://dbpedia.org/ontology/Baronet'),
(rc.BaseballTeam, 'http://dbpedia.org/ontology/BaseballTeam'),
(rc.SportsCoach, 'http://dbpedia.org/ontology/Coach'),
(rc.SportsCoach, 'http://dbpedia.org/ontology/AmericanFootballCoach'),
(rc.SportsCoach, 'http://dbpedia.org/ontology/VolleyballCoach'),
(rc.BasketballLeague, 'http://dbpedia.org/ontology/BaseballLeague'),
(rc.TeamSport, 'http://dbpedia.org/ontology/TeamSport'),
(rc.Parliament, 'http://dbpedia.org/ontology/Parliament'),
(rc.HockeyTeam, 'http://dbpedia.org/ontology/HockeyTeam'),
(rc.Collection, 'http://dbpedia.org/ontology/Type'),
(rc.Beach, 'http://dbpedia.org/ontology/Beach'),
(rc.IrregularMilitaryForce,
'http://dbpedia.org/ontology/MemberResistanceMovement'),
(rc.Tower, 'http://dbpedia.org/ontology/Tower'),
(rc.Database_AbstractContent, 'http://dbpedia.org/ontology/Database'),
(rc.LegalCode, 'http://dbpedia.org/ontology/SystemOfLaw'),
(rc.Lipid, 'http://dbpedia.org/ontology/Lipid'),
(rc.BiologicalTaxon, 'http://dbpedia.org/ontology/Taxon'),
(rc.BiopolymerMolecule, 'http://dbpedia.org/ontology/Biomolecule'),
(rc.BirthEvent, 'http://dbpedia.org/ontology/Birth'),
(rc.CardGame, 'http://dbpedia.org/ontology/CardGame'),
(rc.Meeting_SocialGathering, 'http://dbpedia.org/ontology/Meeting'),
(rc.BloodType, 'http://dbpedia.org/ontology/BloodType'),
(rc.Walkway, 'http://dbpedia.org/ontology/MovingWalkway'),
(rc.Poet, 'http://dbpedia.org/ontology/Poet'),
(rc.Bodybuilder, 'http://dbpedia.org/ontology/Bodybuilder'),
(rc.Psychologist, 'http://dbpedia.org/ontology/Psychologist'),
(rc.Garden, 'http://dbpedia.org/ontology/Garden'),
(rc.NaturalRegion, 'http://dbpedia.org/ontology/NaturalRegion'),
(rc.BoxingSport, 'http://dbpedia.org/ontology/Boxing'),
(rc.BoxingSport, 'http://dbpedia.org/ontology/BoxingCategory'),
(rc.BoxingSport, 'http://dbpedia.org/ontology/BoxingStyle'),
(rc.Prison, 'http://dbpedia.org/ontology/Prison'),
(rc.GovernmentAgency, 'http://dbpedia.org/ontology/GovernmentAgency'),
(rc.BroadcastMediaShow, 'http://dbpedia.org/ontology/Broadcast'),
(rc.NuclearPowerStation, 'http://dbpedia.org/ontology/NuclearPowerStation'),
(rc.ReligiousPerson, 'http://dbpedia.org/ontology/Religious'),
(rc.ComputerFile, 'http://dbpedia.org/ontology/File'),
(rc.BusLine, 'http://dbpedia.org/ontology/BusCompany'),
(rc.MassTransitSystem, 'http://dbpedia.org/ontology/PublicTransitSystem'),
(rc.Tax_COC, 'http://dbpedia.org/ontology/Tax'),
(rc.PopulatedLocality, 'http://dbpedia.org/ontology/Locality'),
(rc.PopulatedLocality, 'http://dbpedia.org/ontology/FrenchLocality'),
(rc.ProfessionalSportsEvent,
'http://dbpedia.org/ontology/NationalFootballLeagueEvent'),
(rc.ConceptualWorkSeries_Periodic,
'http://dbpedia.org/ontology/MultiVolumePublication'),
(rc.CabinetOffice, 'http://dbpedia.org/ontology/GovernmentCabinet'),
(rc.MemorialMarker, 'http://dbpedia.org/ontology/Memorial'),
(rc.CalendarYear, 'http://dbpedia.org/ontology/Year'),
(rc.Camera, 'http://dbpedia.org/ontology/Camera'),
(rc.MobilePhone, 'http://dbpedia.org/ontology/MobilePhone'),
(rc.HumanResidenceArea, 'http://dbpedia.org/ontology/Agglomeration'),
(rc.Canal, 'http://dbpedia.org/ontology/Canal'),
(rc.PlayboyModel, 'http://dbpedia.org/ontology/PlayboyPlaymate'),
(rc.FactoryBuilding, 'http://dbpedia.org/ontology/Factory'),
(rc.Valley, 'http://dbpedia.org/ontology/Valley'),
(rc.Cape_Topographical, 'http://dbpedia.org/ontology/Cape'),
(rc.CarRaceTrack, 'http://dbpedia.org/ontology/Racecourse'),
(rc.RaceTrack, 'http://dbpedia.org/ontology/RaceTrack'),
(rc.RomanCatholicCardinal, 'http://dbpedia.org/ontology/Cardinal'),
(rc.Cartoon, 'http://dbpedia.org/ontology/Cartoon'),
(rc.Cartoon, 'http://dbpedia.org/ontology/HollywoodCartoon'),
(rc.CartoonCharacter, 'http://dbpedia.org/ontology/ComicsCharacter'),
(rc.CartoonCharacter, 'http://dbpedia.org/ontology/AnimangaCharacter'),
(rc.CartoonCharacter, 'http://dbpedia.org/ontology/DisneyCharacter'),
(rc.CartoonCharacter, 'http://dbpedia.org/ontology/NarutoCharacter'),
(rc.Cartoonist, 'http://dbpedia.org/ontology/ComicsCreator'),
(rc.Casino, 'http://dbpedia.org/ontology/Casino'),
(rc.Castle, 'http://dbpedia.org/ontology/Castle'),
(rc.Catalog, 'http://dbpedia.org/ontology/ArtistDiscography'),
(rc.Caterer_Person, 'http://dbpedia.org/ontology/Caterer'),
(rc.Church_Building, 'http://dbpedia.org/ontology/Church'),
(rc.HorsebackRiding, 'http://dbpedia.org/ontology/HorseRiding'),
(rc.Celebrity, 'http://dbpedia.org/ontology/Celebrity'),
(rc.Celebrity_Political, 'http://dbpedia.org/ontology/PoliticianSpouse'),
(rc.CelestialBody, 'http://dbpedia.org/ontology/CelestialBody'),
(rc.Polysaccharide, 'http://dbpedia.org/ontology/Polysaccharide'),
(rc.Mollusk, 'http://dbpedia.org/ontology/Mollusca'),
(rc.Cycad, 'http://dbpedia.org/ontology/Cycad'),
(rc.MusicalComposition_Classical,
'http://dbpedia.org/ontology/ClassicalMusicComposition'),
(rc.Politician, 'http://dbpedia.org/ontology/Politician'),
(rc.Tunnel, 'http://dbpedia.org/ontology/Tunnel'),
(rc.Tunnel, 'http://dbpedia.org/ontology/RailwayTunnel'),
(rc.Tunnel, 'http://dbpedia.org/ontology/RoadTunnel'),
(rc.Tunnel, 'http://dbpedia.org/ontology/WaterwayTunnel'),
(rc.HolyRomanEmperor, 'http://dbpedia.org/ontology/RomanEmperor'),
(rc.Chart, 'http://dbpedia.org/ontology/ChartsPlacements'),
(rc.Infographic, 'http://dbpedia.org/ontology/ElectionDiagram'),
(rc.Chef, 'http://dbpedia.org/ontology/Chef'),
(rc.UNESCOWorldHertitageSite,
'http://dbpedia.org/ontology/SiteOfSpecialScientificInterest'),
(rc.UNESCOWorldHertitageSite,
'http://dbpedia.org/ontology/WorldHeritageSite'),
(rc.Minister_Governmental, 'http://dbpedia.org/ontology/VicePrimeMinister'),
(rc.Marriage_Agreement, 'http://dbpedia.org/ontology/Marriage'),
(rc.Divorce, 'http://dbpedia.org/ontology/Divorce'),
(rc.Christianity, 'http://dbpedia.org/ontology/ChristianDoctrine'),
(rc.Historian, 'http://dbpedia.org/ontology/Historian'),
(rc.Historian, 'http://dbpedia.org/ontology/Egyptologist'),
(rc.LocalReligiousCongregation, 'http://dbpedia.org/ontology/Parish'),
(rc.MovingImage, 'http://dbpedia.org/ontology/MovingImage'),
(rc.Mayor, 'http://dbpedia.org/ontology/Mayor'),
(rc.TradeUnion, 'http://dbpedia.org/ontology/TradeUnion'),
(rc.Satellite, 'http://dbpedia.org/ontology/ArtificialSatellite'),
(rc.Comedian, 'http://dbpedia.org/ontology/Comedian'),
(rc.Comedian, 'http://dbpedia.org/ontology/Humorist'),
(rc.CoalField_Developed, 'http://dbpedia.org/ontology/CoalPit'),
(rc.College, 'http://dbpedia.org/ontology/College'),
(rc.CollegeCoach, 'http://dbpedia.org/ontology/CollegeCoach'),
(rc.PropositionalConceptualWork_DramaGenre,
'http://dbpedia.org/ontology/Drama'),
(rc.ComicalMode, 'http://dbpedia.org/ontology/Comic'),
(rc.ComedyTeam, 'http://dbpedia.org/ontology/ComedyGroup'),
(rc.ComicBookSeries, 'http://dbpedia.org/ontology/Comics'),
(rc.ComicBookSeries, 'http://dbpedia.org/ontology/Manhua'),
(rc.ComicBookSeries, 'http://dbpedia.org/ontology/Manhwa'),
(rc.ComicStrip, 'http://dbpedia.org/ontology/ComicStrip'),
(rc.InternationalOrganization,
'http://dbpedia.org/ontology/InternationalOrganisation'),
(rc.ConflictEvent, 'http://dbpedia.org/ontology/MilitaryConflict'),
(rc.ComplexQuantityPredicate,
'http://dbpedia.org/ontology/GrossDomesticProduct'),
(rc.ComplexQuantityPredicate,
'http://dbpedia.org/ontology/GrossDomesticProductPerCapita'),
(rc.Executive_PublicOfficial, 'http://dbpedia.org/ontology/Lieutenant'),
(rc.Linguist, 'http://dbpedia.org/ontology/Linguist'),
(rc.ConcentrationCamp, 'http://dbpedia.org/ontology/ConcentrationCamp'),
(rc.Convention_Meeting, 'http://dbpedia.org/ontology/Convention'),
(rc.Contest, 'http://dbpedia.org/ontology/Contest'),
(rc.Continent, 'http://dbpedia.org/ontology/Continent'),
(rc.TimeInterval, 'http://dbpedia.org/ontology/TimePeriod'),
(rc.TimeInterval, 'http://dbpedia.org/ontology/YearInSpaceflight'),
(rc.Insurgency, 'http://dbpedia.org/ontology/Rebellion'),
(rc.CountySeat, 'http://dbpedia.org/ontology/CountrySeat'),
(rc.CourtCase_Appellate,
'http://dbpedia.org/ontology/SupremeCourtOfTheUnitedStatesCase'),
(rc.Statistics, 'http://dbpedia.org/ontology/Statistic'),
(rc.MusicalPerformer, 'http://dbpedia.org/ontology/ClassicalMusicArtist'),
(rc.MusicalPerformer, 'http://dbpedia.org/ontology/MusicalArtist'),
(rc.Crater, 'http://dbpedia.org/ontology/Crater'),
(rc.Crater, 'http://dbpedia.org/ontology/LunarCrater'),
(rc.Creek, 'http://dbpedia.org/ontology/Creek'),
(rc.CricketField, 'http://dbpedia.org/ontology/CricketGround'),
(rc.CricketTeam, 'http://dbpedia.org/ontology/CricketTeam'),
(rc.SystemOfWrittenMaterials, 'http://dbpedia.org/ontology/LiteraryGenre'),
(rc.Fern, 'http://dbpedia.org/ontology/Fern'),
(rc.PoliticalAction, 'http://dbpedia.org/ontology/PoliticalFunction'),
(rc.Demographics, 'http://dbpedia.org/ontology/Demographics'),
(rc.DepthPerception, 'http://dbpedia.org/ontology/Depth'),
(rc.Mine_ExcavationFacility, 'http://dbpedia.org/ontology/Mine'),
(rc.DigitalCamera, 'http://dbpedia.org/ontology/DigitalCamera'),
(rc.Dike, 'http://dbpedia.org/ontology/Dike'),
(rc.Diploma, 'http://dbpedia.org/ontology/Diploma'),
(rc.Prefecture, 'http://dbpedia.org/ontology/Prefecture'),
(rc.Ginkgo, 'http://dbpedia.org/ontology/Ginkgo'),
(rc.Vein, 'http://dbpedia.org/ontology/Vein'),
(rc.DwarfStar, 'http://dbpedia.org/ontology/BrownDwarf'),
(rc.King_HeadOfState, 'http://dbpedia.org/ontology/PolishKing'),
(rc.PyramidMonument, 'http://dbpedia.org/ontology/Pyramid'),
(rc.ElementStuff, 'http://dbpedia.org/ontology/ChemicalElement'),
(rc.Embryology, 'http://dbpedia.org/ontology/Embryology'),
(rc.Skyscraper, 'http://dbpedia.org/ontology/Skyscraper'),
(rc.Employer, 'http://dbpedia.org/ontology/Employer'),
(rc.Zoologist, 'http://dbpedia.org/ontology/Entomologist'),
(rc.PopulationOfType_Organism_Whole,
'http://dbpedia.org/ontology/Population'),
(rc.Escalator, 'http://dbpedia.org/ontology/Escalator'),
(rc.Train, 'http://dbpedia.org/ontology/Train'),
(rc.Planet, 'http://dbpedia.org/ontology/Planet'),
(rc.ExurbanNeighborhood, 'http://dbpedia.org/ontology/Intercommunality'),
(rc.SoccerCompetition, 'http://dbpedia.org/ontology/SoccerTournament'),
(rc.WrestlingSportsEvent, 'http://dbpedia.org/ontology/WrestlingEvent'),
(rc.GenreTypes, 'http://dbpedia.org/ontology/Genre'),
(rc.Farmer, 'http://dbpedia.org/ontology/Farmer'),
(rc.Guitar, 'http://dbpedia.org/ontology/Guitar'),
(rc.FilmFestival, 'http://dbpedia.org/ontology/FilmFestival'),
(rc.Flooding, 'http://dbpedia.org/ontology/StormSurge'),
(rc.ForeignOffice, 'http://dbpedia.org/ontology/OverseasDepartment'),
(rc.FormulaOneRacer, 'http://dbpedia.org/ontology/FormulaOneRacer'),
(rc.FortTheShelterRegion, 'http://dbpedia.org/ontology/Fort'),
(rc.WineTypeByRegion,
'http://dbpedia.org/ontology/ControlledDesignationOfOriginWine'),
(rc.TVShowHost, 'http://dbpedia.org/ontology/TelevisionHost'),
(rc.GatedCommunity, 'http://dbpedia.org/ontology/GatedCommunity'),
(rc.SocialGroup, 'http://dbpedia.org/ontology/Group'),
(rc.GeneralManager_SportsTeam, 'http://dbpedia.org/ontology/SportsManager'),
(rc.GeneralManager_SportsTeam, 'http://dbpedia.org/ontology/SoccerManager'),
(rc.GeographicalDirection_General,
'http://dbpedia.org/ontology/CardinalDirection'),
(rc.Prehistoric, 'http://dbpedia.org/ontology/PrehistoricalPeriod'),
(rc.HotSpring, 'http://dbpedia.org/ontology/HotSpring'),
(rc.GnetopsidaClass, 'http://dbpedia.org/ontology/Gnetophytes'),
(rc.Musical, 'http://dbpedia.org/ontology/Musical'),
(rc.GolfTournament, 'http://dbpedia.org/ontology/GolfTournament'),
(rc.StateGovernor, 'http://dbpedia.org/ontology/Governor'),
(rc.Grape, 'http://dbpedia.org/ontology/Grape'),
(rc.GraveMarker, 'http://dbpedia.org/ontology/GraveMonument'),
(rc.GreenAlgae, 'http://dbpedia.org/ontology/GreenAlga'),
(rc.Mill_ManufacturingFacility, 'http://dbpedia.org/ontology/Mill'),
(rc.Guitarist, 'http://dbpedia.org/ontology/Guitarist'),
(rc.Play, 'http://dbpedia.org/ontology/Play'),
(rc.Organ_MusicalInstrument, 'http://dbpedia.org/ontology/Organ'),
(rc.HeraldicDevice, 'http://dbpedia.org/ontology/Blazon'),
(rc.HistoricBuilding, 'http://dbpedia.org/ontology/HistoricBuilding'),
(rc.RailwayTramwayCar, 'http://dbpedia.org/ontology/TrainCarriage'),
(rc.HospitalBuilding, 'http://dbpedia.org/ontology/Hospital'),
(rc.HumanTypeByLifeStageType, 'http://dbpedia.org/ontology/PersonalEvent'),
(rc.ShoppingMallBuilding, 'http://dbpedia.org/ontology/ShoppingMall'),
(rc.InformationManagementUse,
'http://dbpedia.org/ontology/InformationAppliance'),
(rc.MassCommunicating, 'http://dbpedia.org/ontology/Media'),
(rc.InternetMovieDatabase, 'http://dbpedia.org/ontology/Imdb'),
(rc.RoadwayJunction, 'http://dbpedia.org/ontology/RoadJunction'),
(rc.TelevisionStation, 'http://dbpedia.org/ontology/TelevisionStation'),
(rc.Jockey, 'http://dbpedia.org/ontology/Jockey'),
(rc.Polyhedron, 'http://dbpedia.org/ontology/Polyhedron'),
(rc.MartialArtsSportsEvent,
'http://dbpedia.org/ontology/MixedMartialArtsEvent'),
(rc.StillImage, 'http://dbpedia.org/ontology/StillImage'),
(rc.LawFirm, 'http://dbpedia.org/ontology/LawFirm'),
(rc.Ligament, 'http://dbpedia.org/ontology/Ligament'),
(rc.LycophytinaSubdivision, 'http://dbpedia.org/ontology/ClubMoss'),
(rc.LymphFluid, 'http://dbpedia.org/ontology/Lymph'),
(rc.Matador, 'http://dbpedia.org/ontology/BullFighter'),
(rc.MathematicalThing, 'http://dbpedia.org/ontology/MathematicalConcept'),
(rc.PornStar, 'http://dbpedia.org/ontology/Pornstar'),
(rc.MediaOrganization, 'http://dbpedia.org/ontology/Broadcaster'),
(rc.MediaOrganization, 'http://dbpedia.org/ontology/BroadcastNetwork'),
(rc.MedicalDatabase, 'http://dbpedia.org/ontology/BiologicalDatabase'),
(rc.ParliamentMember, 'http://dbpedia.org/ontology/MemberOfParliament'),
(rc.Monastery_Residence, 'http://dbpedia.org/ontology/Monastery'),
(rc.Sculptor, 'http://dbpedia.org/ontology/Sculptor'),
(rc.Novel, 'http://dbpedia.org/ontology/Novel'),
(rc.Satellite_HeavenlyBody, 'http://dbpedia.org/ontology/Satellite'),
(rc.Mosque, 'http://dbpedia.org/ontology/Mosque'),
(rc.MotorRacing, 'http://dbpedia.org/ontology/MotorRace'),
(rc.Motorcyclist_Professional, 'http://dbpedia.org/ontology/MotocycleRacer'),
(rc.Motorcyclist_Professional, 'http://dbpedia.org/ontology/MotorcycleRider'),
(rc.Motorcyclist_Professional, 'http://dbpedia.org/ontology/SpeedwayRider'),
(rc.MusicDirector, 'http://dbpedia.org/ontology/MusicDirector'),
(rc.MusicFestival, 'http://dbpedia.org/ontology/MusicFestival'),
(rc.NASCARDriver, 'http://dbpedia.org/ontology/NascarDriver'),
(rc.OlympicCompetition, 'http://dbpedia.org/ontology/OlympicEvent'),
(rc.OlympicCompetition, 'http://dbpedia.org/ontology/OlympicResult'),
(rc.NationalAnthem, 'http://dbpedia.org/ontology/NationalAnthem'),
(rc.NewspaperSeries, 'http://dbpedia.org/ontology/Newspaper'),
(rc.NobelPrize, 'http://dbpedia.org/ontology/NobelPrize'),
(rc.NordicCombinedSportsEvent, 'http://dbpedia.org/ontology/NordicCombined'),
(rc.Note_Document, 'http://dbpedia.org/ontology/Annotation'),
(rc.Novella, 'http://dbpedia.org/ontology/LightNovel'),
(rc.ObjectTypeByStyle, 'http://dbpedia.org/ontology/Fashion'),
(rc.Opera, 'http://dbpedia.org/ontology/Opera'),
(rc.Orphan, 'http://dbpedia.org/ontology/Orphan'),
(rc.Playwright, 'http://dbpedia.org/ontology/PlayWright'),
(rc.Pope, 'http://dbpedia.org/ontology/Pope'),
(rc.PopulatedPlacePart, 'http://dbpedia.org/ontology/SubMunicipality'),
(rc.Windmill, 'http://dbpedia.org/ontology/Windmill'),
(rc.PresidentOfOrganization, 'http://dbpedia.org/ontology/President'),
(rc.PrimeMinister_HeadOfGovernment,
'http://dbpedia.org/ontology/PrimeMinister'),
(rc.ProWrestler, 'http://dbpedia.org/ontology/SumoWrestler'),
(rc.Producer, 'http://dbpedia.org/ontology/Producer'),
(rc.University, 'http://dbpedia.org/ontology/University'),
(rc.PublicWaterSystem, 'http://dbpedia.org/ontology/DistrictWaterBoard'),
(rc.Publisher, 'http://dbpedia.org/ontology/Publisher'),
(rc.Songwriter, 'http://dbpedia.org/ontology/SongWriter'),
(rc.RadioShow, 'http://dbpedia.org/ontology/RadioProgram'),
(rc.RadioStation_Organization, 'http://dbpedia.org/ontology/RadioStation'),
(rc.RadioTalkShowHost, 'http://dbpedia.org/ontology/RadioHost'),
(rc.TalkShowHost, 'http://dbpedia.org/ontology/Host'),
(rc.TalkShowHost, 'http://dbpedia.org/ontology/Presenter'),
(rc.Railway, 'http://dbpedia.org/ontology/RailwayLine'),
(rc.Referee, 'http://dbpedia.org/ontology/Referee'),
(rc.RestArea, 'http://dbpedia.org/ontology/RestArea'),
(rc.Resume, 'http://dbpedia.org/ontology/Resume'),
(rc.RocketEngine, 'http://dbpedia.org/ontology/RocketEngine'),
(rc.Streetcar, 'http://dbpedia.org/ontology/Tram'),
(rc.SabreFencer, 'http://dbpedia.org/ontology/Fencer'),
(rc.Saint, 'http://dbpedia.org/ontology/Saint'),
(rc.Screenwriter, 'http://dbpedia.org/ontology/ScreenWriter'),
(rc.ServiceStation, 'http://dbpedia.org/ontology/FillingStation'),
(rc.Shrine_Religious, 'http://dbpedia.org/ontology/Shrine'),
(rc.SkiArea, 'http://dbpedia.org/ontology/SkiArea'),
(rc.SkiResort_Attraction, 'http://dbpedia.org/ontology/SkiResort'),
(rc.SolarEclipse, 'http://dbpedia.org/ontology/SolarEclipse'),
(rc.SpaceShuttle, 'http://dbpedia.org/ontology/SpaceShuttle'),
(rc.SpaceStation, 'http://dbpedia.org/ontology/SpaceStation'),
(rc.SubwayStation, 'http://dbpedia.org/ontology/MetroStation'),
(rc.Synagogue, 'http://dbpedia.org/ontology/Synagogue'),
(rc.TVCharacter, 'http://dbpedia.org/ontology/SoapCharacter'),
(rc.TVSeason, 'http://dbpedia.org/ontology/TelevisionSeason'),
(rc.TVShow_Single, 'http://dbpedia.org/ontology/TelevisionEpisode'),
(rc.TeamHandballPlayer, 'http://dbpedia.org/ontology/HandballPlayer'),
(rc.TelevisionDirector, 'http://dbpedia.org/ontology/TelevisionDirector'),
(rc.TelevisionStar, 'http://dbpedia.org/ontology/TelevisionPersonality'),
(rc.TheHumanLifeCycle, 'http://dbpedia.org/ontology/LifeCycleEvent'),
(rc.TheatreDirector, 'http://dbpedia.org/ontology/TheatreDirector'),
(rc.TimeInPosition_Served, 'http://dbpedia.org/ontology/TermOfOffice'),
(rc.ToDoList, 'http://dbpedia.org/ontology/TrackList'),
(rc.TransformerSubstation,
'http://dbpedia.org/ontology/ElectricalSubstation'),
(rc.Treadmill, 'http://dbpedia.org/ontology/Treadmill'),
(rc.USRepresentative, 'http://dbpedia.org/ontology/Congressman'),
(rc.VicePresidentOfOrganization, 'http://dbpedia.org/ontology/VicePresident'),
(rc.ViticulturalDomain, 'http://dbpedia.org/ontology/WineRegion'),
(rc.WaterPoweredMill, 'http://dbpedia.org/ontology/Watermill'),
(rc.WaterTower, 'http://dbpedia.org/ontology/WaterTower'),
(rc.WaterwayLock, 'http://dbpedia.org/ontology/Lock'),
(rc.WindPoweredTurbine, 'http://dbpedia.org/ontology/WindMotor'),
(rc.Winery, 'http://dbpedia.org/ontology/Winery'),
(rc.WomensTennisAssociationTournament,
'http://dbpedia.org/ontology/WomensTennisAssociationTournament'),
(rc.WorkQuantity, 'http://dbpedia.org/ontology/UnitOfWork'),
(rc.ZooOrganization, 'http://dbpedia.org/ontology/Zoo'),
(kko.TopicsCategories, 'http://dbpedia.org/ontology/TopicalConcept'),
(kko.Eukaryotes, 'http://dbpedia.org/ontology/Eukaryote'),
(kko.Geopolitical, 'http://dbpedia.org/ontology/AdministrativeRegion')]
Then, we can decompose those results to construct up our format for annotating a s-p-o tuple to get the exact mapping predicate used:
list(kko.mappingAsObject[rc.Currency, rc.dbpedia_ontology_id, 'http://dbpedia.org/ontology/Currency'])
['owl:equivalentClass']
With some string replacements and shifting the orders, we then can see how we can get a reconstruction of the input rows we used to load the external mappings in the first place, with our example becoming:
rc.Currency, owl:equivalentClass, http://dbpedia.org/ontology/Currency
(Using ‘rc.’ rather than base IRI in this example.) For the moment, we will defer writing the full routine, but will eventually add it to our extract.py
module. We will also embed this code block into a loop that writes our individual mapping files to disk, thereby completing the external mapping portion of our roundtrip.
*.ipynb
file. It may take a bit of time for the interactive option to load.